Member-only story
Top Python Interview Problems
Part 1: Arrays & Hash Tables, Heap, Two Pointer, Intervals, Linked List, Binary Search, Sliding Window Technique, Strings, Greedy Algorithms, and Dynamic Programming.
This article will be useful for those preparing for software engineering roles as well as machine learning engineer roles. At most companies we will have to clear a coding interview involving data structures and CS algorithms. Also, in this series of articles we will first cover the topics in breadth before we re-visit for depth. It’s better to first get an idea of all the types of problems, this way when you see a new problem you can figure out which data structure and algorithm is suitable to solve a problem.
1. Arrays and Hash Tables
Problem¹: Given an array of integers nums(assume positive) and an integer target, return indices of the two numbers such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice. You can return the answer in any order.
Example: Input: nums = [2,7,11,15], target = 26. Output: [2,3]
Solution:
Explanation: When we says arrays, in Python it’s nothing but a list. First, look at the brute force solution — it has a nested 2 for loops which iterates over all numbers in the array twice hence a worst time complexity of O(n²). Note that I am giving a simpler explanation of time complexity and any time you have a nested for loops you can safely assume that it’s not the optimal solution and you will get rejected if you can only come up with this solution in an interview.
Input: nums = [2,7,11,15], target = 26
Why j starts at i + 1? We are avoiding redundant calculations as addition is commutative for real numbers and also we don’t need to add same number twice as per problem statement.
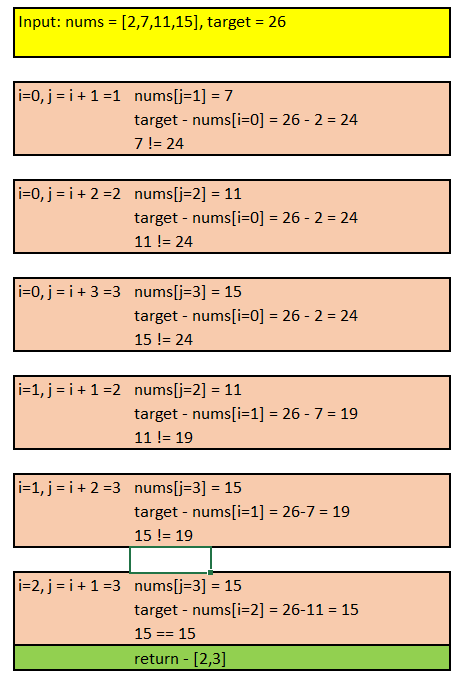